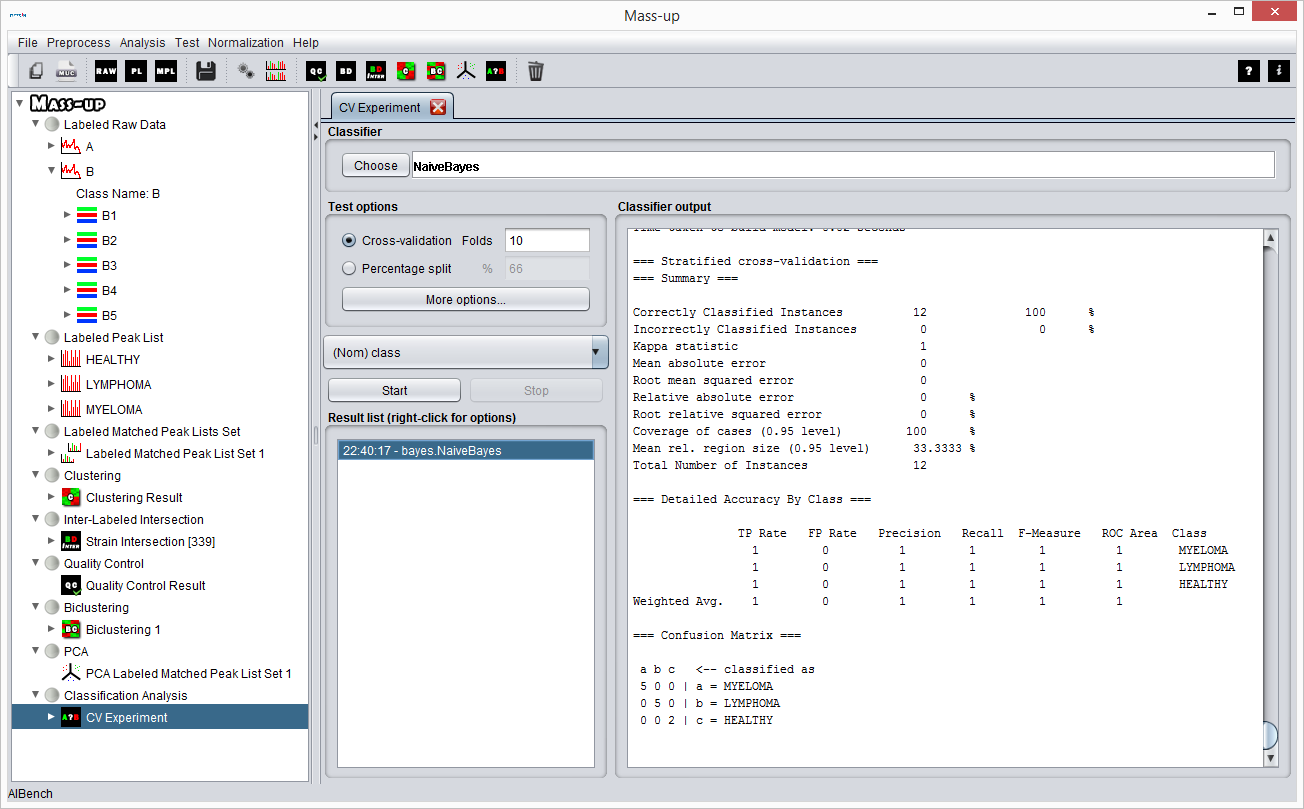
AIBench applications are are designed as a set of Operations, DataTypes and Views, which keeps different concerns well separated.
AIBench generates input dialogs, manages the main window, menu and toolbars, and many more. These tasks are very common and repeatitive. We try to help you as much as we can in order to allow you to center in your application specific features.
AIBench is based on plugins. You can build your application from one or more plugins, allowing you maximize your code reuse.
AIBench can be customized in many ways: application layout, icons, help, welcome screen, custom components, etc. You can also work with the API to create very specific behaviours.
All operations performed while the user is using the final application generate a script that is a "macro" allowing you to create (and edit) an unattended pipeline.
AIBench is a Java application framework, a leading language with a huge set of libraries for almost anything. In addition, AIBench is 100% integrated with Maven. You can create an application starting from the archetype.
AIBench follows the IPO (Input-Process-Output) model as its conceptual vision.
Your application can be seen as a set of processes that take data as input and produces data as output.
Each data can be seen with a viewer.
The user will interacts with the application by requesting process executions, as well as datatype exploration through viewers.
@Operation(description = "this operation adds two numbers")
public class Sum {
private int x, y;
@Port(direction = Direction.INPUT, name = "x param")
public void setX(int x) {
this.x = x;
}
@Port(direction = Direction.INPUT, name = "y param")
public void setY(int y) {
this.y = y;
}
@Port(direction = Direction.OUTPUT)
public int sum() {
return this.x + this.y;
}
}
Operations are the key components of AIBench applications, implementing processes. An Operation is a Java class defining a set of inputs and outputs (ports).
The operation in the example will generate an input dialog automatically
Datatypes can be raw Java classes (POJO), without any annotation.
However, you can define the internals of your object in order to make AIBench aware of its subparts, allowing you to use these parts in Operations or displaying them in Views.
@Datatype(structure=Structure.COMPLEX)
class AComplex {
...
private AList subpart;
...
@Clipboard(name = "subpart1")
public AList getSubpart(){
return subElems;
}
@Clipboard(name = "subpart2")
public String getSubpart2(){
return "hello";
}
@Property(name = "simple field")
public int simpleField(){
return 75;
}
}
Views are custom components displaying Datatype instances.
Start your application is very easy. You need Java and Maven, and then run the following command:
# Create application
mvn archetype:generate -DarchetypeGroupId=es.uvigo.ei.sing \
-DarchetypeArtifactId=aibench-archetype -DarchetypeVersion=2.10.2 \
-DgroupId=es.uvigo.ei.sing -DartifactId=my-aibench-application \
-DinteractiveMode=false \
-DarchetypeCatalog=http://sing.ei.uvigo.es/maven2/archetype-catalog.xml
# Build
cd my-aibench-application
mvn package
# Run
cd target/dist
sh run.sh